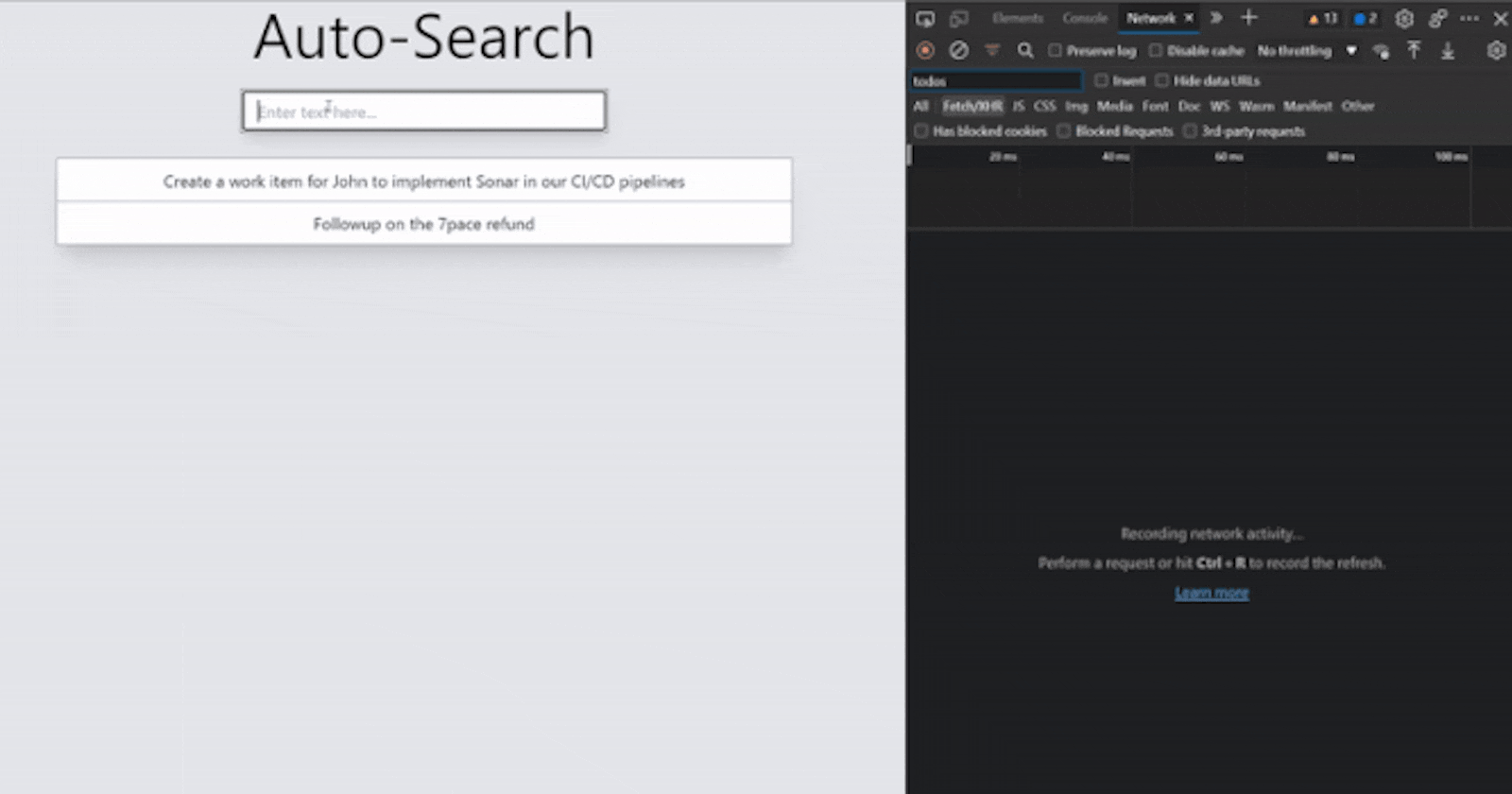
How-to implement auto-search in Angular (aka Angular debounce)?
Are you having trouble implementing autosearch or simply debouncing an input in Angular? Here's a quick guide on how you should do it.
If you’ve ever had to implement a simple search functionality, the chances are that you would’ve been asked to convert that to an autosearch as well. Tbh, implementing autosearch (with a debounce) in Angular is rather simple and there are quite a few articles out there already. Unfortunately, most — if not all — of those articles use the fromEvent() function of RxJS which requires accessing the native element from within your Angular component, something that I like to avoid unless absolutely necessary.
In this article, I’ll show you how-to implement autosearch with a debounce only using Angular bindings, RxJS subjects and RxJS debounce. First, lets understand the basic steps involved in autosearch (and incase you’re already familiar, you can skip to the code below).
- User enters text into the search bar (input field).
- We wait x seconds before performing the search(this is referred to as debounce).
- We show the search results.
Now, let’s code this! First of all, we’ll create the following properties & functions in our *.component.ts file.
public searchText: string;
private readonly \_autoSearch$: Subject<string> = new Subject<string>();
private readonly \_debounce: number = 500;
ngOnInit(): void {
this.todos$ = this.\_autoSearch$.pipe(
debounceTime(this.\_debounce),
distinctUntilChanged(),
switchMap((text) => {
// TODO: REPLACE THIS WITH YOUR OWN HTTP CALL HERE.
return this.\_http.get<ITodo\[\]>(\`[http://www.some-api.com/todos?search=${text}\`](http://www.some-api.com/todos?search=$%7Btext%7D`));
})
);
}
public onSearch(text: string) {
this.\_autoSearch$.next(text);
}
So, here’s what's going on in the code above:
- We’ve created two properties: searchText (a string) for storing the user’s input and _autoSearch$ (a RxJS Subject) for binding to the JavaScript keyup event.
- We also subscribed to the _autoSearch$ subject, with a debounce of 500ms, in Angular’s OnInit lifecycle hook to make sure that when the user enters text, we wait 500ms before we catch that and perform our search API call.
Once you’ve done that, lets just add the following HTML into your \.component.html* file.
<input
placeholder="Enter text here..."
type="search"
(keyup)="onSearch(searchText)"
\[(ngModel)\]="searchText"
/>
And you’re done! You just created an input field and bound its value to the searchText property and the keyup event to our onSearch function. You should now be able to let you users autosearch as they enter text into the search bar.
If you have any confusions or question, you can reach out to me in the comments below or you can check this out in action on StackBlitz.
Happy Coding!
This article is a part of my Coding series. You may find other useful articles for your daily development there as well.
If you found this helpful or enjoyed reading the article, please do the following:
- Click the clap 👏 (or any other emoji) on the right so that others can see this too
- Follow me on Twitter (@thehaseebahm3d)
- Buy me a coffee to show your support